【WordPress】記事集を表示するショートコード
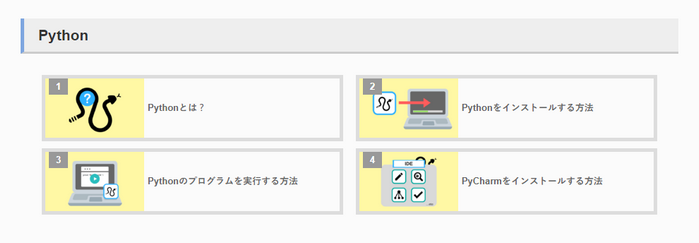
Beproで記事集を簡単に作れるようにしたかったので作成した。
作り方
記事集のコードを取得するクラスを定義する
下記のクラスをPHPファイルに定義する。
クラス内に定義されている定数のTHUMBNAIL_WIDTH
とTHUMBNAIL_HEIGHT
には任意のサムネイルサイズを指定する。
class PostCollection {
const THUMBNAIL_NAME = 'post-collection';
const THUMBNAIL_WIDTH = 140;
const THUMBNAIL_HEIGHT = 84;
private function __construct() {}
public static function get_posts_code( $ids ) {
if ( $ids === '' ) return;
$datas = self::get_post_datas( $ids );
if ( !$datas->have_posts() ) return;
$code = '';
$code .= '<ol class="post-collection">';
while ( $datas->have_posts() ) {
$code .= self::get_post_code( $datas );
}
$code .= '</ol>';
return $code;
}
private static function get_post_datas( $ids ) {
$conditions = [
'post__in' => $ids,
'orderby' => 'post__in',
'order' => 'ASC',
'ignore_sticky_posts' => 1,
'posts_per_page' => -1,
];
return new WP_Query( $conditions );
}
private static function get_post_code( $datas ) {
$datas->the_post();
$permalink = esc_url( get_the_permalink() );
$code = <<< EOT
<li class="post">
<a href="$permalink">
<div class="inner">
EOT;
$code .= self::get_thumbnail_or_no_image_code();
$code .= self::get_post_title_code();
$code .= '</div></a></li>';
return $code;
}
private static function get_thumbnail_or_no_image_code() {
return has_post_thumbnail() ?
self::get_thumbnail_code() :
self::get_no_image_code()
;
}
private static function get_thumbnail_code() {
return get_the_post_thumbnail( null, self::THUMBNAIL_NAME );
}
private static function get_no_image_code() {
$width = self::THUMBNAIL_WIDTH;
$height = self::THUMBNAIL_HEIGHT;
return <<< EOT
<div class="no-image" style="width: {$width}px; height: {$height}px;">
NO IMAGE
</div>
EOT;
}
private static function get_post_title_code() {
$title = esc_html( get_the_title() );
return sprintf( '<span class="title">%s</span>', $title );
}
}
サムネイルのサイズを追加する
after_setup_theme
アクションフックを用いて、サムネイルのサイズを追加する。
( ※ 既にメディアに入っている画像は、追加したサイズのサムネイルが作られていないため再作成が必要となる。 )
function setup_theme() {
add_theme_support( 'post-thumbnails' );
add_image_size(
PostCollection::THUMBNAIL_NAME,
PostCollection::THUMBNAIL_WIDTH,
PostCollection::THUMBNAIL_HEIGHT,
true
);
}
add_action( 'after_setup_theme', 'setup_theme' );
ショートコードを追加する
add_shortcode
関数を用いて、ショートコードを追加する。
add_shortcode( 'post-collection', [ 'PostCollection', 'get_posts_code' ] );
使い方
ショートコードを書く
[post-collection]
というショートコードを記述し、引数に「記事のID」を指定する。
[post-collection 1111 2222]
これでその箇所に次のようなHTMLが出力される。( img
要素が長いため大部分は省略した。 )
<ol class="post-collection">
<li class="post">
<a href="http://sample.com/sample/hoge/">
<div class="inner">
<img width="140" height="84" src="http://sample.com/wp-content/uploads/20xx/xx/hoge-140x84.png">
<span class="title">ほげ</span>
</div>
</a>
</li>
<li class="post">
<a href="http://sample.com/sample/fuga/">
<div class="inner">
<div class="no-image" style="width: 140px; height: 84px;">NO IMAGE</div>
<span class="title">ふが</span>
</div>
</a>
</li>
</ol>
CSSで見た目を整える
後は出力されたHTMLの各要素に付いているクラスを用いて、CSSで見た目を整える。